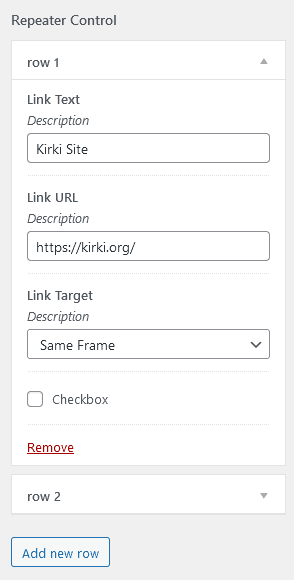
Repeater controls allow you to build repeatable blocks of fields. For example, you can create a set of fields that will contain a checkbox and a textfield. The user will then be able to add “rows”, and each row will contain a checkbox and a textfield.
Supported Fields
The repeater can contain only a limited amount of fields.
- Text
- Select
- Checkbox
- Image
- URL
- Upload
- Dropdown Pages
- Color
- Radio
- Radio-image
- Textarea
Example
Creating a repeater control where each row contains 2 textfields, a select field & a checkbox:
new \Kirki\Field\Repeater(
[
'settings' => 'repeater_setting',
'label' => esc_html__( 'Repeater Control', 'kirki' ),
'section' => 'repeater_section',
'priority' => 10,
'default' => [
[
'link_text' => esc_html__( 'Kirki Site', 'kirki' ),
'link_url' => 'https://kirki.org/',
'link_target' => '_self',
'checkbox' => false,
],
[
'link_text' => esc_html__( 'Kirki WP', 'kirki' ),
'link_url' => 'https://wordpress.org/plugins/kirki/',
'link_target' => '_blank',
'checkbox' => true,
],
],
'fields' => [
'link_text' => [
'type' => 'text',
'label' => esc_html__( 'Link Text', 'kirki' ),
'description' => esc_html__( 'Description', 'kirki' ),
'default' => '',
],
'link_url' => [
'type' => 'text',
'label' => esc_html__( 'Link URL', 'kirki' ),
'description' => esc_html__( 'Description', 'kirki' ),
'default' => '',
],
'link_target' => [
'type' => 'select',
'label' => esc_html__( 'Link Target', 'kirki' ),
'description' => esc_html__( 'Description', 'kirki' ),
'default' => '_self',
'choices' => [
'_blank' => esc_html__( 'New Window', 'kirki' ),
'_self' => esc_html__( 'Same Frame', 'kirki' ),
],
],
'checkbox' => [
'type' => 'checkbox',
'label' => esc_html__( 'Checkbox', 'kirki' ),
'default' => false,
],
],
]
);
Creating a repeater control where the label has a dynamic name based on a field’s input. This will use ['row_label']['value']
if nothing is returned from the specified field:
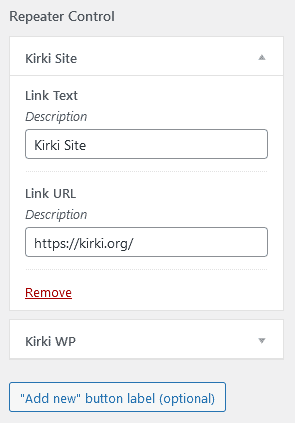
new \Kirki\Field\Repeater(
[
'settings' => 'repeater_setting_2',
'label' => esc_html__( 'Repeater Control', 'kirki' ),
'section' => 'section_id',
'priority' => 10,
'row_label' => [
'type' => 'field',
'value' => esc_html__( 'Your Custom Value', 'kirki' ),
'field' => 'link_text',
],
'button_label' => esc_html__( '"Add new" button label (optional) ', 'kirki' ),
'default' => [
[
'link_text' => esc_html__( 'Kirki Site', 'kirki' ),
'link_url' => 'https://kirki.org/',
],
[
'link_text' => esc_html__( 'Kirki WP', 'kirki' ),
'link_url' => 'https://wordpress.org/plugins/kirki/',
],
],
'fields' => [
'link_text' => [
'type' => 'text',
'label' => esc_html__( 'Link Text', 'kirki' ),
'description' => esc_html__( 'Description', 'kirki' ),
'default' => '',
],
'link_url' => [
'type' => 'text',
'label' => esc_html__( 'Link URL', 'kirki' ),
'description' => esc_html__( 'Description', 'kirki' ),
'default' => '',
],
],
]
);
To limit the amount of rows, simply add the choices
argument and pass a limit as shown here:
'choices' => [
'limit' => 3
]
Usage
<?php
// Default values for 'my_repeater_setting' theme mod.
$defaults = [
[
'link_text' => esc_html__( 'Kirki Site', 'kirki' ),
'link_url' => 'https://kirki.org/',
],
[
'link_text' => esc_html__( 'Kirki Repository', 'kirki' ),
'link_url' => 'https://github.com/aristath/kirki',
],
];
// Theme_mod settings to check.
$settings = get_theme_mod( 'my_repeater_setting', $defaults );
?>
<div class="kirki-links">
<?php foreach ( $settings as $setting ) : ?>
<a href="<?php $setting['link_url']; ?>">
<?php $setting['link_text']; ?>
</a>
<?php endforeach; ?>
</div>